Have you ever had an idea that could be executed using JavaScript, but there is no browser extension available for that purpose? In this post, I will show how anything that can be done by Javascript can be achieved with bookmarklets on browsers that allow users to save JavaScript functions as bookmarks.
What is a bookmarklet?
A bookmarklet is a small snippet of JavaScript code that can be stored as a bookmark in a web browser. When clicked, the bookmarklet executes the JavaScript code, which can add new features to the browser or perform specific tasks on the current web page.
Bookmarklets are similar to browser extensions, but they are simpler and do not require installation.
Following are some applications of bookmarklets:
- Extract data from a web page.
- Analyze on-page SEO elements and provide a summary of key insights.
- Extract and display meta tags, heading structure, and other SEO-related information.
- Count the number of words on a webpage, helpful for content analysis.
- Highlight or list images without alt text, aiding in accessibility checks.
- Display information about the cookies set by the current webpage.
- Assess the readability of the content on a page and provide a readability score.
- Extract color palettes from a webpage and display them in a user-friendly format.
- Change the appearance of a web page
- Search some words from the page on the Internet
- Share the current page on Social media
Remember, the possibilities for bookmarklets are vast, and you can tailor them to your specific needs or interests!
How to create and use a bookmarklet
Follow the steps below to create and use a bookmarklet on your Chromium-based browser.
- Prepare the JavaScript function you need.
- Create a new bookmark in your browser.
- Paste your function as the bookmark URL
- Choose an appropriate name for your bookmarklet
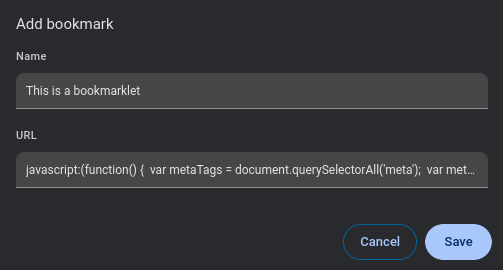
Bookmarklets for auditing pages from an SEO perspective
Since bookmarklets execute JavaScript functions, they can be used for auditing web pages from an SEO point of view. The following are just some ideas for auditing purposes. They can help you develop your ideas for a variety of purposes.
You can paste all the functions below into the Google Chrome console and press “enter” to check its functionality before creating a bookmarklet.
1. Bookmarklet to filter the current page in the Search Console performance report
We can use the PAGE filter in the Google Search Console performance report by “URLs containing” and “Exact URL” filters. The bookmarklet below will use the “URLs containing” filter:
javascript: (function() {
window.open(`https://search.google.com/search-console/performance/search-analytics?resource_id=${encodeURIComponent(window.location.origin)}&page=*${encodeURIComponent(window.location.href)}`)
})();
The bookmarklet below will use the “Exact URL” filter:
javascript: (function() {
window.open(`https://search.google.com/search-console/performance/search-analytics?resource_id=${encodeURIComponent(window.location.origin)}&page=!${encodeURIComponent(window.location.href)}`)
})();
According to the above two bookmarklets, page=!$ is used for the “URLs containing” filter, and page=*$ is used for the “Exact URL” filter.
Access to the “URL Prefix” property in the Google Search Console account linked to the present domain is necessary.
2. Bookmarklet to send the current page to Google Rich Result Testing Tool
Clicking on this bookmarklet will send the current page to the Google Rich Result Testing Tool.
javascript: (function() {
window.open(`https://search.google.com/test/rich-results?url=${encodeURIComponent(window.location.href)}`)
})();
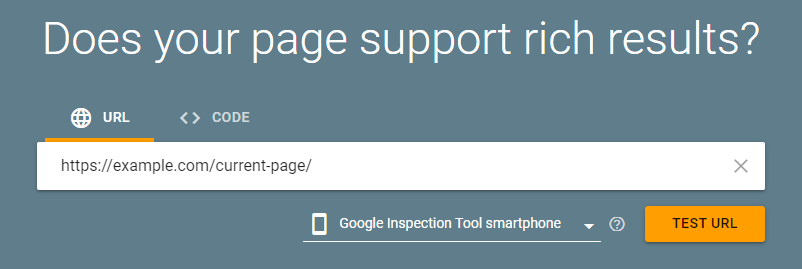
3. Bookmarklet to send the current page to Google AMP Testing Tool
Clicking on this bookmarklet will send the current page to the Google Rich Result Testing Tool.
javascript: (function() {
window.open(`https://search.google.com/test/amp?url=${encodeURIComponent(window.location.href)}`)
})();
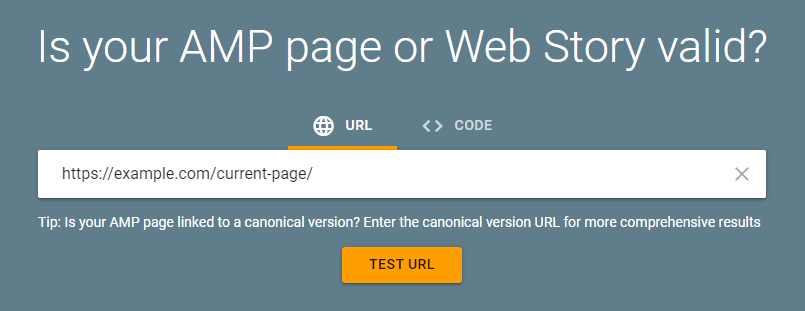
4. Bookmarklet to send the current page to Google PageSpeed Insights
javascript: (function() {
window.open(`https://pagespeed.web.dev/analysis?url=${encodeURIComponent(window.location.href)}`)
})();

5. Bookmarklet to highlight all <a> elements
javascript: (function() {
var anchorElements = document.querySelectorAll('a');
anchorElements.forEach(function(anchor) {
anchor.style.background = 'yellow';
anchor.style.border = '2px solid blue';
anchor.style.padding = '2px';
});
if (anchorElements.length > 0) {
alert('Links highlighted on the page.');
} else {
alert('No anchor elements found on this page.');
}
})();
6. Bookmarklet to show the number of <a> and highlight them
javascript: (function() {
var anchorElements = document.querySelectorAll('a');
anchorElements.forEach(function(anchor, index) {
var backgroundColor = getRandomColor();
anchor.style.background = backgroundColor;
anchor.style.border = '2px solid #000';
anchor.style.padding = '2px';
anchor.setAttribute('data-link-index', index + 1);
});
var totalLinks = anchorElements.length;
if (totalLinks > 0) {
alert('Total links on the page: ' + totalLinks);
} else {
alert('No anchor elements found on this page.');
}
function getRandomColor() {
return '#' + Math.floor(Math.random() * 16777215).toString(16);
}
})();
7. Bookmarklet to show Alt attribute of images on them
javascript: (function() {
var images = document.querySelectorAll('img');
images.forEach(function(img) {
var altText = img.alt || 'N/A';
var altOverlay = document.createElement('div');
altOverlay.style.position = 'absolute';
altOverlay.style.top = '0';
altOverlay.style.left = '0';
altOverlay.style.background = 'rgba(255, 255, 255, 0.9)';
altOverlay.style.padding = '5px';
altOverlay.style.border = '1px solid #ccc';
altOverlay.style.zIndex = '9999';
altOverlay.textContent = 'Alt Text: ' + altText;
img.style.position = 'relative';
img.parentNode.insertBefore(altOverlay, img);
});
})();
8. Bookmarklet to show every heading type beside all <h>s
javascript:(function() {
var headings = document.querySelectorAll('h1, h2, h3, h4, h5, h6');
headings.forEach(function(heading) {
var headingTypeContainer = document.createElement('div');
headingTypeContainer.style = 'position: relative; display: inline-block; margin-left: 10px;';
var headingTypeLabel = document.createElement('div');
headingTypeLabel.style = 'position: absolute; top: -20px; right: 0; background-color: #000; color: #fff; padding: 5px;border-radius:5px;';
headingTypeLabel.textContent = heading.tagName.toLowerCase();
headingTypeContainer.appendChild(headingTypeLabel);
heading.appendChild(headingTypeContainer);
});
})();
9. Bookmarklet to show the canonical address and meta robots of the current page
javascript:(function() {
function getMetaContentByName(name) {
var metaTag = document.querySelector('meta[name="' + name + '"]');
return metaTag ? metaTag.getAttribute('content') : null;
}
var canonicalUrl = getMetaContentByName('canonical');
var canonicalMessage = canonicalUrl ? 'Canonical URL:' + '\n' + canonicalUrl : 'Canonical URL does not exist! π€';
var metaRobots = getMetaContentByName('robots');
var robotsMessage = metaRobots ? 'Meta Robots:' + '\n' + metaRobots : 'Meta Robots does not exist! π€';
alert(canonicalMessage + '\n' + '\n' + robotsMessage);
})();
10. Bookmarklet to show all preconnect, dns-prefetch, preload, and prefetch of the current page
javascript:(function() {
function getLinkInfo(linkType) {
var linkElements = document.querySelectorAll('link[rel="' + linkType + '"]');
var linkCount = linkElements.length;
if (linkCount > 0) {
var linkInfo = Array.from(linkElements).map(function(link) {
return link.href;
}).join('\n');
alert(linkType + ' links (' + linkCount + '):\n' + linkInfo);
} else {
alert('No ' + linkType + ' links found on this page! π€');
}
}
getLinkInfo('preconnect');
getLinkInfo('dns-prefetch');
getLinkInfo('preload');
getLinkInfo('prefetch');
})();
11. Bookmarklet to show the number of some elements of the page
According to the bookmarklet below, you can add or remove elements with regard to your needs.
javascript:(function() {
function countElements(tagName) {
return document.querySelectorAll(tagName).length;
}
var totalElementsCount = document.querySelectorAll('*').length;
var h1Count = countElements('h1');
var h2Count = countElements('h2');
var h3Count = countElements('h3');
var h4Count = countElements('h4');
var h5Count = countElements('h5');
var h6Count = countElements('h6');
var divCount = countElements('div');
var pCount = countElements('p');
var aCount = countElements('a');
var spanCount = countElements('span');
var imgCount = countElements('img');
var pictureCount = countElements('picture');
var videoCount = countElements('video');
var scriptCount = countElements('script');
var alertMessage = `
Total Number of Elements: ${totalElementsCount}
Number of Specific Elements:
<h1>: ${h1Count} | <h2>: ${h2Count} | <h3>: ${h3Count}
<h4>: ${h4Count} | <h5>: ${h5Count} | <h6>: ${h6Count}
<div>: ${divCount} | <p>: ${pCount} | <span>: ${spanCount} | <a>: ${aCount}
<img>: ${imgCount} | <picture>: ${pictureCount} | <video>: ${videoCount}
<scripts>: ${scriptCount}
`;
alert(alertMessage);
})();
12. Bookmarklet to extract Schema Markups and show them on a new tab
javascript:(function() {
function extractJsonLd() {
const jsonLdScripts = document.querySelectorAll('script[type="application/ld+json"]');
const extractedData = [];
jsonLdScripts.forEach(script => {
try {
const json = JSON.parse(script.textContent);
extractedData.push(json);
} catch (error) {
console.error('Error parsing JSON-LD:', error);
}
});
if (extractedData.length > 0) {
const jsonString = JSON.stringify(extractedData, null, 2);
const highlightedJsonString = jsonString.replace(/(".*schema\.org.*")/g, '<span style="background-color: lightgreen;">$1</span>');
const blob = new Blob([highlightedJsonString], { type: 'text/html' });
const objectURL = URL.createObjectURL(blob);
const newTab = window.open();
newTab.document.write('<pre>' + highlightedJsonString + '</pre>');
newTab.document.close();
} else {
console.log('No JSON-LD Schema Markup found on this page.');
}
}
extractJsonLd();
})();
13. Bookmarklet to open the current domain on archive.org
javascript:(function() {
var currentDomain = window.location.origin;
var waybackURL = 'https://web.archive.org/web/*/' + decodeURIComponent(currentDomain);
window.open(waybackURL, '_blank');
})();
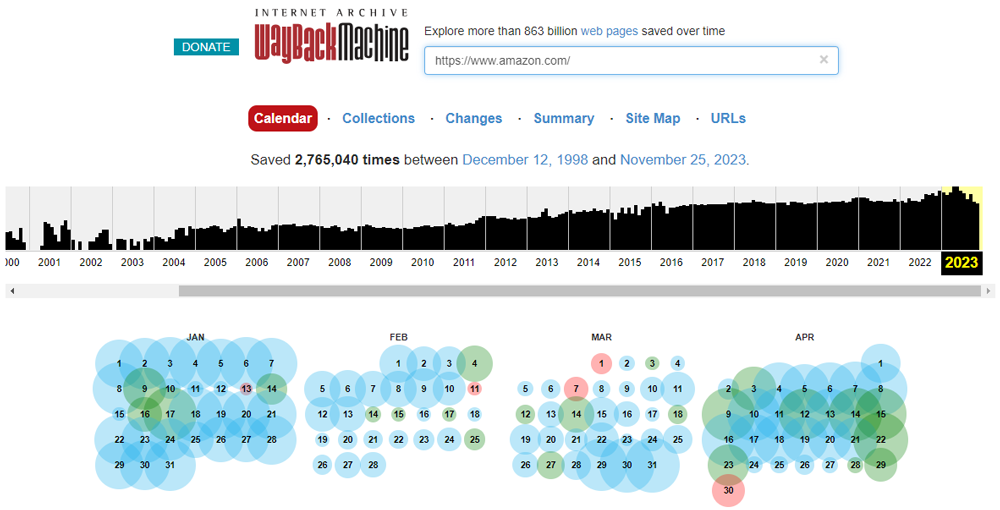
14. Bookmarklet to display information about the cookies set by the current webpage
Clicking on this bookmarklet will display information about cookies set by the current webpage in a modal.
This example presents basic cookie information and may not cover more complex scenarios or cookies set through client-side scripts.
javascript:(function() {
function createCookieModal() {
var modalContainer = document.createElement('div');
modalContainer.style.position = 'fixed';
modalContainer.style.top = '50%';
modalContainer.style.left = '50%';
modalContainer.style.transform = 'translate(-50%, -50%)';
modalContainer.style.backgroundColor = 'white';
modalContainer.style.padding = '20px';
modalContainer.style.border = '1px solid #222';
modalContainer.style.boxShadow = '0 0 10px rgba(0, 0, 0, 0.3)';
modalContainer.style.zIndex = '9999';
modalContainer.style.width = '1000px';
modalContainer.style.direction = 'ltr';
modalContainer.style.textAlign = 'left';
modalContainer.style.wordWrap = 'break-word';
var closeButton = document.createElement('button');
closeButton.textContent = 'Close';
closeButton.style.padding = '5px 10px';
closeButton.style.marginTop = '10px';
closeButton.style.cursor = 'pointer';
closeButton.style.right = '10px';
closeButton.style.top = '10px';
closeButton.style.position = 'fixed';
closeButton.addEventListener('click', function() {
modalContainer.style.display = 'none';
});
var cookies = document.cookie.split(';');
for (var i = 0; i < cookies.length; i++) {
var cookieLine = document.createElement('div');
cookieLine.textContent = cookies[i].trim();
modalContainer.appendChild(cookieLine);
}
modalContainer.appendChild(closeButton);
document.body.appendChild(modalContainer);
}
createCookieModal();
})();
15. Bookmarklet to count the number of words within a specific element with the selector .entry-content
When you click on the bookmarklet while on a webpage, it will display an alert with the word count within the element with the selector .entry-content. Note that this is a basic example and may not cover all scenarios, especially if the content is dynamically loaded or if there are other considerations for word counting.
javascript:(function() {
var entryContent = document.querySelector('.entry-content');
function countWords(text) {
var words = text.split(/\s+/).filter(function(word) {
return word.length > 0;
});
return words.length;
}
function showWordCount() {
if (entryContent) {
var contentText = entryContent.textContent || entryContent.innerText;
var wordCount = countWords(contentText);
alert('Word Count in .entry-content: ' + wordCount);
} else {
alert('No element with class .entry-content found on this page.');
}
}
showWordCount();
})();
Here are two bookmarklets for fun π
16. Bookmarklet to show a running man π and a running πββοΈ at the bottom of the page
javascript:(function() {
function createRunningEmoji(emoji) {
var runningElement = document.createElement('div');
runningElement.innerHTML = emoji;
runningElement.style.cssText = 'position: fixed; bottom: 0px; font-size: 60px; animation: run-animation 10s linear infinite;';
document.body.appendChild(runningElement);
}
function animateRunningWoman() {
setTimeout(function() {
createRunningEmoji('π');
}, 2000);
}
createRunningEmoji('πββοΈ');
animateRunningWoman();
var style = document.createElement('style');
style.textContent = `
@keyframes run-animation {
0% { right: 0; }
100% { right: calc(100vw - 20px); }
}
`;
document.head.appendChild(style);
})();
17. Bookmarklet to show some random emojis that are rotating and falling from top of the page
javascript: (function() {
var emojis = ['π', 'π', 'π€', 'π', 'π', 'π', 'πΎ', 'π€£', 'π₯³', 'πΊ', 'β€οΈ'];
var emojiContainer = document.createElement('div');
emojiContainer.style.cssText = 'position: fixed; top: 0; left: 0; pointer-events: none; z-index: 1000;';
function createEmoji() {
var emojiElement = document.createElement('div');
var randomEmoji = emojis[Math.floor(Math.random() * emojis.length)];
emojiElement.innerHTML = randomEmoji;
emojiElement.style.cssText = 'position: absolute; font-size: 40px; opacity: 0.8;';
emojiElement.style.left = Math.random() * window.innerWidth + 'px';
emojiElement.style.animation = 'falling-emoji 5s linear infinite';
emojiContainer.appendChild(emojiElement);
}
function animateEmojis() {
setInterval(function() {
createEmoji();
}, 3000);
}
document.body.appendChild(emojiContainer);
var style = document.createElement('style');
style.textContent = ` @keyframes falling-emoji { 0% { transform: translateY(0) rotate(0deg); } 100% { transform: translateY(calc(100vh - 20px)) rotate(360deg); } } `;
document.head.appendChild(style);
createEmoji();
animateEmojis();
})();
How to debug bookmarklets
You can verify the code by opening the into your web browser’s console and pasting the code into it. If the code runs without any errors, the issue is likely located elsewhere.
Ensure that the bookmarklet possesses the required permissions.
FAQs about bookmarklets
A bookmark is a saved link to a specific webpage, allowing quick access to that page with a single click. On the other hand, a bookmarklet is a bookmarked JavaScript code snippet that, when clicked, performs a specific action or function on the current webpage.
Yes, you can use bookmarklets on mobile devices’ browsers that support JavaScript
π GitHub